-
Notifications
You must be signed in to change notification settings - Fork 8.2k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
## Summary Closes #23303 ~(@cqliu1 can you confirm this too?)~ confirmed Fixes the way we capture the request info when configuring the socket and providing it to plugins via `callWithRequest`. Instead of exposing a route that returns the info, simply use the request object that comes back from `server.inject`. Also adds a check in the `elasticsearchClient` handler exposed to plugins to ensure the session is still valid because using `callWithRequest`. 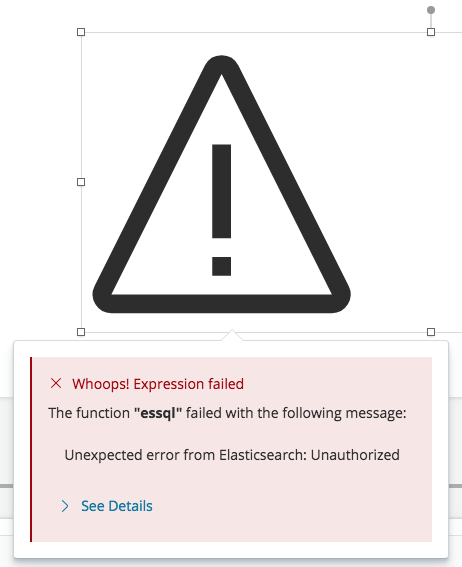 *Note:* the actual error message is a bit different, but this is how the failure is exposed to the user
- Loading branch information
Showing
7 changed files
with
147 additions
and
77 deletions.
There are no files selected for viewing
103 changes: 103 additions & 0 deletions
103
x-pack/plugins/canvas/server/lib/__tests__/create_handlers.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,103 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import expect from 'expect.js'; | ||
import { createHandlers } from '../create_handlers'; | ||
|
||
let securityMode = 'pass'; | ||
const authError = new Error('auth error'); | ||
|
||
const mockRequest = { | ||
headers: 'i can haz headers', | ||
}; | ||
|
||
const mockServer = { | ||
plugins: { | ||
security: { | ||
authenticate: () => ({ | ||
succeeded: () => (securityMode === 'pass' ? true : false), | ||
error: securityMode === 'pass' ? null : authError, | ||
}), | ||
}, | ||
elasticsearch: { | ||
getCluster: () => ({ | ||
callWithRequest: (...args) => Promise.resolve(args), | ||
}), | ||
}, | ||
}, | ||
config: () => ({ | ||
has: () => false, | ||
get: val => val, | ||
}), | ||
info: { | ||
uri: 'serveruri', | ||
}, | ||
}; | ||
|
||
describe('server createHandlers', () => { | ||
let handlers; | ||
|
||
beforeEach(() => { | ||
securityMode = 'pass'; | ||
handlers = createHandlers(mockRequest, mockServer); | ||
}); | ||
|
||
it('provides helper methods and properties', () => { | ||
expect(handlers).to.have.property('environment', 'server'); | ||
expect(handlers).to.have.property('serverUri'); | ||
expect(handlers).to.have.property('httpHeaders', mockRequest.headers); | ||
expect(handlers).to.have.property('elasticsearchClient'); | ||
}); | ||
|
||
describe('elasticsearchClient', () => { | ||
it('executes callWithRequest', async () => { | ||
const [request, endpoint, payload] = await handlers.elasticsearchClient( | ||
'endpoint', | ||
'payload' | ||
); | ||
expect(request).to.equal(mockRequest); | ||
expect(endpoint).to.equal('endpoint'); | ||
expect(payload).to.equal('payload'); | ||
}); | ||
|
||
it('rejects when authentication check fails', () => { | ||
securityMode = 'fail'; | ||
return handlers | ||
.elasticsearchClient('endpoint', 'payload') | ||
.then(() => { | ||
throw new Error('elasticsearchClient should fail when authentication fails'); | ||
}) | ||
.catch(err => { | ||
// note: boom pre-pends error messages with "Error: " | ||
expect(err.message).to.be.equal(`Error: ${authError.message}`); | ||
}); | ||
}); | ||
|
||
it('works without security', async () => { | ||
// create server without security plugin | ||
const mockServerClone = { | ||
...mockServer, | ||
plugins: { ...mockServer.plugins }, | ||
}; | ||
delete mockServerClone.plugins.security; | ||
expect(mockServer.plugins).to.have.property('security'); // confirm original server object | ||
expect(mockServerClone.plugins).to.not.have.property('security'); | ||
|
||
// this shouldn't do anything | ||
securityMode = 'fail'; | ||
|
||
// make sure the method still works | ||
handlers = createHandlers(mockRequest, mockServerClone); | ||
const [request, endpoint, payload] = await handlers.elasticsearchClient( | ||
'endpoint', | ||
'payload' | ||
); | ||
expect(request).to.equal(mockRequest); | ||
expect(endpoint).to.equal('endpoint'); | ||
expect(payload).to.equal('payload'); | ||
}); | ||
}); | ||
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
21 changes: 0 additions & 21 deletions
21
x-pack/plugins/canvas/server/routes/get_auth/get_auth_header.js
This file was deleted.
Oops, something went wrong.
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters